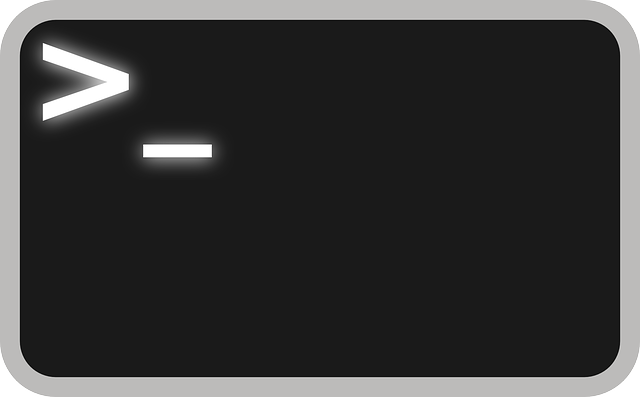
This is not strictly Swift or SwiftUI related, but I was excited about it nonetheless.
In yesterday’s Daily Coding Tip I said I prefer GUIs to CLIs, so I thought I’d challenge myself to do a tutorial entirely via Terminal.
I usually refuse Xcode’s offer to put new projects under source control, because I’m often experimenting and don’t necessarily want a record of early prototypes.
But it’s surprisingly complicated to get that project that has no source control to the point where it not only has local commits but has also been pushed to a remote repository.
Until now…
Installing The GitHub CLI With Homebrew
If you haven’t installed Homebrew already, open Terminal and paste this command:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Once you have Homebrew, run this to install the GitHub CLI:
brew install gh
Run this to log into the GitHub CLI once installed:
gh auth login
I used HTTPS and authenticated with a browser, but you may choose differently.
Adding The GitHub CLI Alias
This command will add the only alias we need for the GitHub CLI:
gh alias set new 'repo create --private --push --source=.'
This simply creates a private remote repository and pushes whatever it finds in the current folder.
Running the gh new
command will create the remote and push your commit(s) if you have a local repository already. If you don’t have a local repository the command will fail, citing the fact that the directory is not yet a git repository.
Adding The Git Alias
Run the following command to add a new Git alias to your global ~/.gitconfig
file:
git config --global alias.new '!new() { cd -- ${GIT_PREFIX:-.}; if getopts "f:" arg; then mkdir "$OPTARG"; cd "$OPTARG"; fi; touch README.md; git init; git add .; git commit -m "Initial commit"; }; new'
The exclamation point (!) allows the use of non-git commands, but it also changes the default location of the shell to the user’s home folder.
To fix this we simply change directory to GIT_PREFIX:-.
, which is wherever the git command is being called from.
git new
This new alias allows you to create a new local repository in the current folder, regardless of whether it contains files or is empty.
git new -f MyNewProject
Running this command creates a new subfolder called MyNewProject
.
The subfolder argument is passed as $OPTARG
, as it is the first argument after the -f
option letter. If the -f
option is not passed then any other arguments are ignored and the command is run the same way as git new
in the current folder without creating a subfolder.
Combining the aliases
I’ve thought about various ways to combine the aliases into one, calling them one after another, but I think doing everything at once is probably overkill in a lot of situations.
Let’s say I want to create a new subfolder with a local repo and then push it to a new GitHub remote:
git new -f MyNewProject && cd MyNewProject && gh new
git new
returns me to the directory where I first called the command, meaning I need to manually enter the subfolder if I created one.
If I don’t want to create a subfolder, and instead want to create a new repository in the current folder, I simply run this:
git new && gh new
If you really want to add this combination as a Z-Shell alias, run this:
echo -n 'alias newgit="git new && gh new"' >> ~/.zshrc; source ~/.zshrc
Just bear in mind that this newgit
alias can only be called in the folder you want to use it.
You can’t pass it a subfolder name because it will still run gh new
in the original folder and not the subfolder.