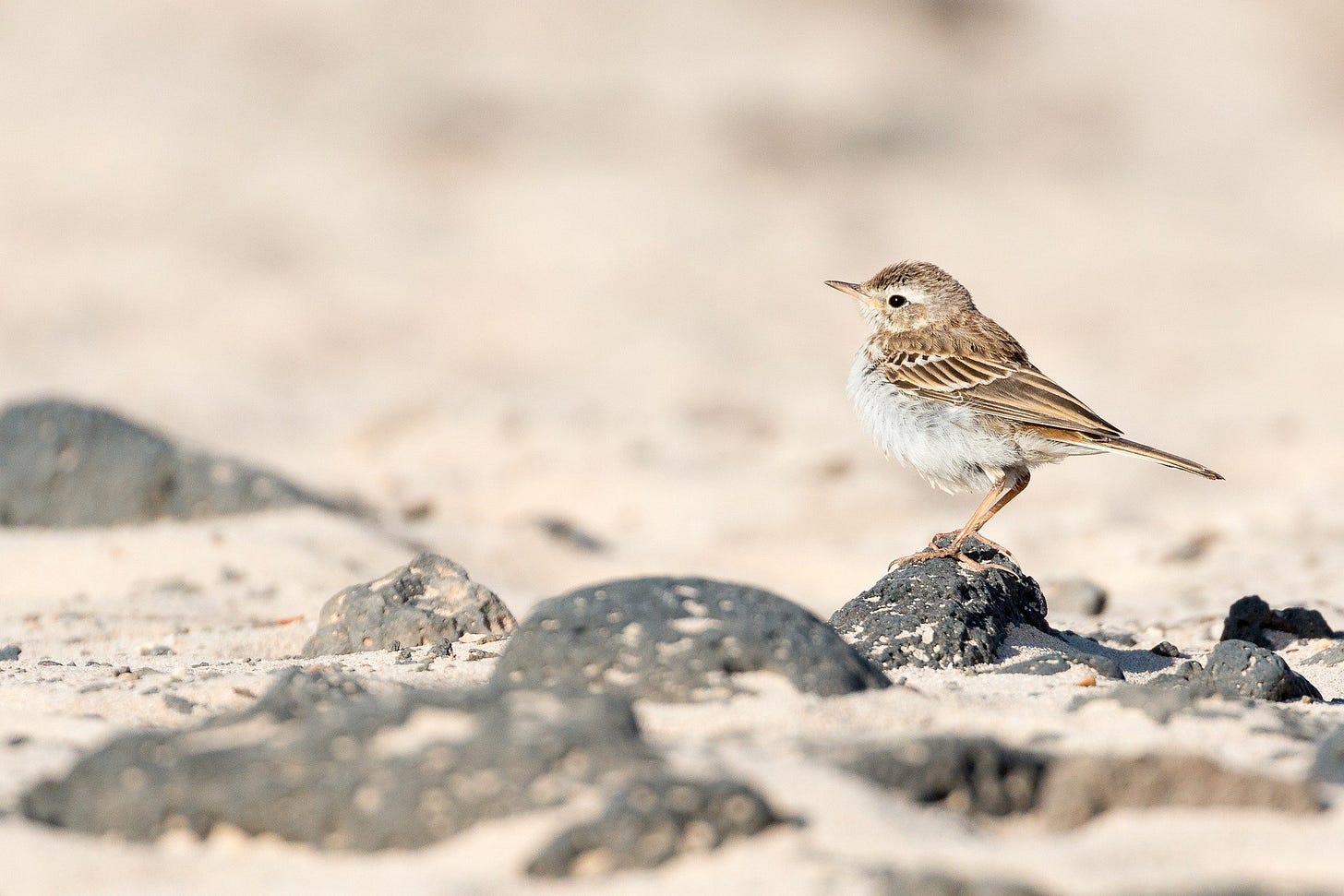
You might have never wondered what happens when you add a question mark at the end of a type. This is actually a shorthand for an enumeration that takes a generic type and, as you might have guessed, it’s called Optional
. It has two cases: .none
has no associated value, and .some
has an associated value of the type you choose. You can still construct optionals the long-handed way, although the only reason I can think of is to illustrate how it works as I am doing now.
In my first example, optionalString0
, I use the shorthand to specify the String?
type. When you don’t want an optional you can use type inference, which assumes what type you want from the literal you initialise it with:
let text = “Hello” //text is inferred to be of type String
You can’t do this with optionals. You need to specify you want an optional string, otherwise you won’t get one. You especially can’t use a nil
literal with type inference, as Swift has no idea what your underlying type should be. In my second example, optionalString1
, I do the longhanded version of the same thing. The question mark in optionalString0
tells Swift that I want an Optional<String>
, without me having to write it that way.
Next we get to the enum cases. As you can see in optionalString2
and optionalString3
, you can either set it to .none
or .some
.
You cannot set it to .some
without an associated value but that makes sense.
Finally I end with optionals that have an initial value. The last one is interesting, as I didn’t realise it was possible to initialise an Optional
directly. To make things even more interesting, I actually made it an optional optional. As you can see in the preview on the right, it ends up getting printed as Optional(Optional(“Hello”))
. I’m not exactly sure why you’d want to wrap an optional in an optional, but it’s fascinating that you need to unwrap each optional separately with the unsafe ! operator (or another safer method).
If you’re wondering what the unsafelyUnwrapped
property is, it’s not quite the same as the ! operator.
To quote Apple’s documentation:
The
unsafelyUnwrapped
property provides the same value as the forced unwrap operator (postfix!
). However, in optimized builds (-O
), no check is performed to ensure that the current instance actually has a value. Accessing this property in the case of anil
value is a serious programming error and could lead to undefined behavior or a runtime error.
Consider yourself warned!